In SQL Server, the comparison operators are used to test for equality and inequality. These operators are used in the WHERE clause to determine which records to select.
Following is a list of the SQL Server comparison operators:
Index | Comparison Operator | Description |
---|---|---|
1) | = | It specifies equal symbol. |
2) | <> | It specifies not equal symbol. |
3) | != | It specifies not equal symbol. |
4) | > | It specifies greater than symbol. |
5) | >= | It specifies greater than or equal symbol. |
6) | < | It specifies less than symbol. |
7) | <= | It specifies less than or equal symbol. |
8) | !> | It specifies not greater than symbol. |
9) | !< | It specifies not less than symbol. |
10) | IN ( ) | It matches a value in a list. |
11) | NOT | It is used to negate a condition. |
12) | BETWEEN | It is used to specify within a range (inclusive) value. |
13) | IS NULL | It specifies null value. |
14) | IS NOT NULL | It specifies non-null value. |
15) | LIKE | It specifies pattern matching with % and _ |
16) | EXISTS | It specifies that the condition is met if subquery returns at least one row. |
Equality Operator
In SQL Server database, Equality Operator “=” is used to test for equality in a query.
Example:
We have a table named “Employees”, having the following data:
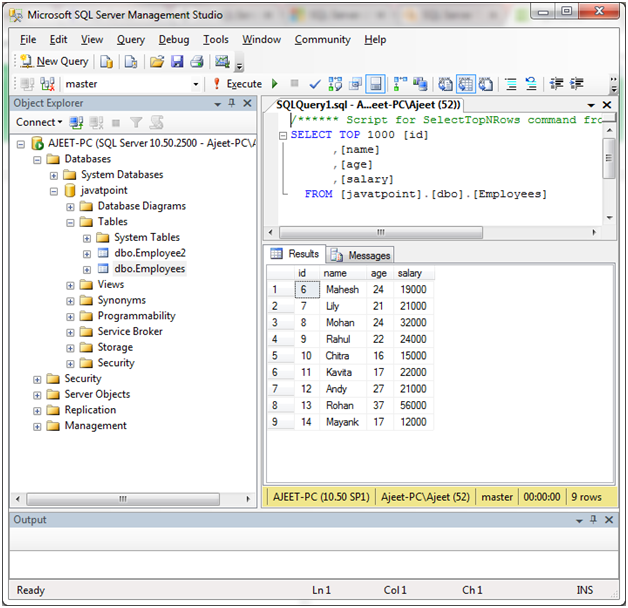
Use the following query to select the specific data where “name” = “Lily”:
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE name = 'Lily';
Output:
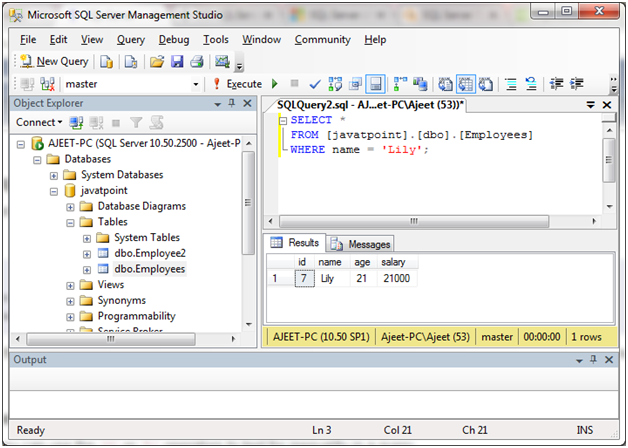
Inequality Operator
In SQL Server, inequality operators “<> or !=” are used to test for inequality in a query.
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE name <> 'Lily';
Output:
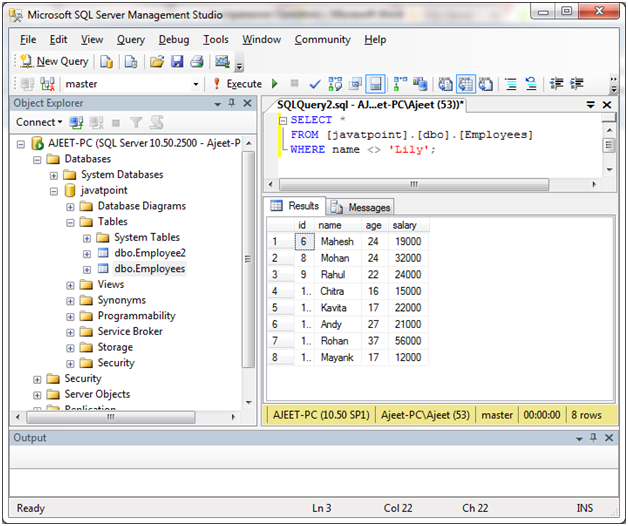
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE name != 'Lily';
Output:
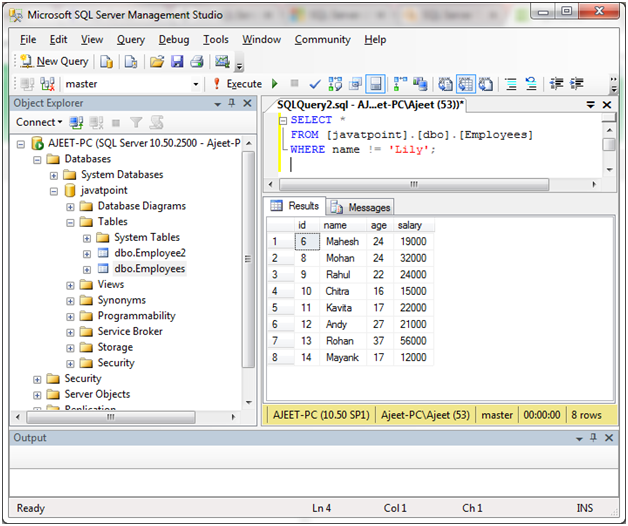
Greater Than Operator
Greater Than “>” Operator is used to test for an expression that it is “greater than”.
Example:
Let’s select employees from the table “Employees” where salary > 15000.
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE salary > 15000;
Output:
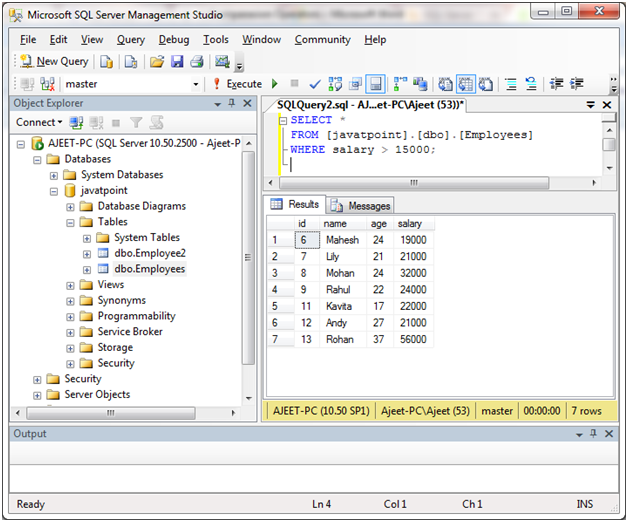
Greater Than or Equal Operator
Greater Than or Equal “>=” Operator is used to test for an expression that it is “greater than or equal to”.
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE salary >= 15000;
Output:
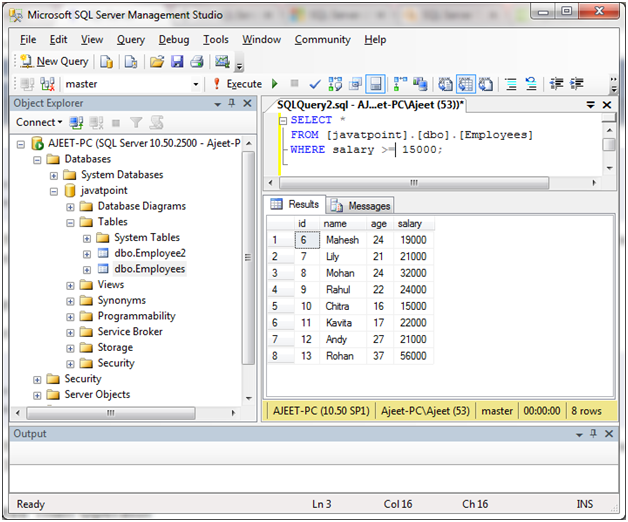
Less than Operator
Less Than “<” Operator is used to test for an expression that it is “less than” the other one.
Example:
Select all employees from the table “Employees” where salary is < 20000.
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE salary < 20000;
Output:
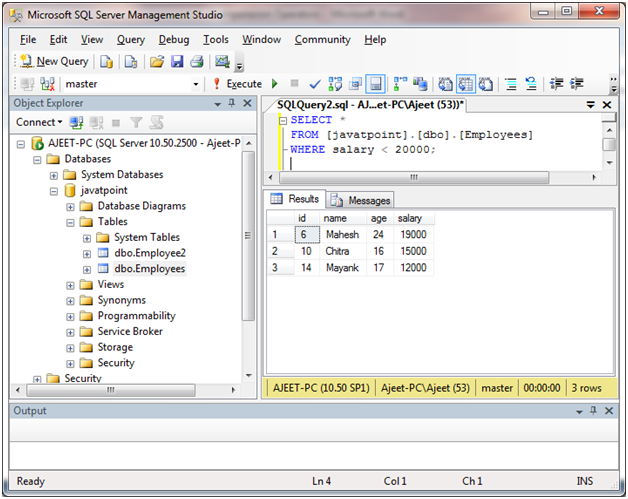
Less Than or Equal Operator
Less Than or Equal “<=” Operator is used to test for an expression that it is “less than or equal to” the other one.
SELECT *
FROM [javatpoint].[dbo].[Employees]
WHERE salary <= 20000;
Output:
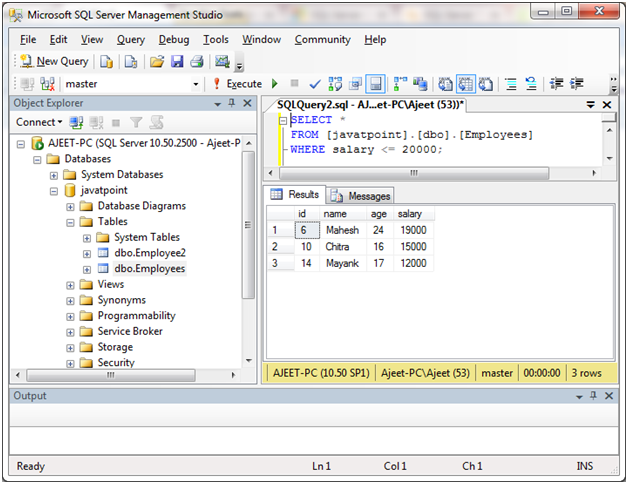
Leave a Reply