In JavaScript, a cookie can contain only a single name-value pair. However, to store more than one name-value pair, we can use the following approach: –
- Serialize the custom object in a JSON string, parse it and then store in a cookie.
- For each name-value pair, use a separate cookie.
Examples to Store Name-Value pair in a Cookie
Example 1
Let’s see an example to check whether a cookie contains more than one name-value pair.
<!DOCTYPE html>
<html>
<head>
</head>
<body>
Name: <input type="text" id="name"><br>
Email: <input type="email" id="email"><br>
Course: <input type="text" id="course"><br>
<input type="button" value="Set Cookie" onclick="setCookie()">
<input type="button" value="Get Cookie" onclick="getCookie()">
<script>
function setCookie()
{
//Declaring 3 key-value pairs
var info="Name="+ document.getElementById("name").value+";Email="+document.getElementById("email").value+";Course="+document.getElementById("course").value;
//Providing all 3 key-value pairs to a single cookie
document.cookie=info;
}
function getCookie()
{
if(document.cookie.length!=0)
{
//Invoking key-value pair stored in a cookie
alert(document.cookie);
}
else
{
alert("Cookie not available")
}
}
</script>
</body>
</html>
Output:
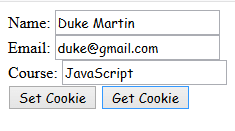
On clicking Get Cookie button, the below dialog box appears.

Here, we can see that only a single name-value is displayed.
However, if you click, Get Cookie without filling the form, the below dialog box appears.

Example 2
Let’s see an example to store different name-value pairs in a cookie using JSON.
<!DOCTYPE html>
<html>
<head>
</head>
<body>
Name: <input type="text" id="name"><br>
Email: <input type="email" id="email"><br>
Course: <input type="text" id="course"><br>
<input type="button" value="Set Cookie" onclick="setCookie()">
<input type="button" value="Get Cookie" onclick="getCookie()">
<script>
function setCookie()
{
var obj = {};//Creating custom object
obj.name = document.getElementById("name").value;
obj.email = document.getElementById("email").value;
obj.course = document.getElementById("course").value;
//Converting JavaScript object to JSON string
var jsonString = JSON.stringify(obj);
document.cookie = jsonString;
}
function getCookie()
{
if( document.cookie.length!=0)
{
//Parsing JSON string to JSON object
var obj = JSON.parse(document.cookie);
alert("Name="+obj.name+" "+"Email="+obj.email+" "+"Course="+obj.course);
}
else
{
alert("Cookie not available");
}
}
</script>
</body>
</html>
Output:
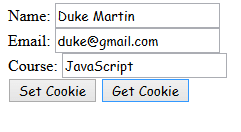
On clicking Get Cookie button, the below dialog box appears.
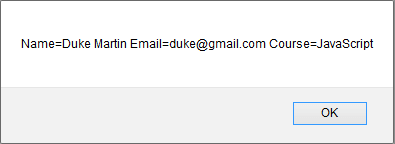
Here, we can see that all the stored name-value pairs are displayed.
Example 3
Let’s see an example to store each name-value pair in a different cookie.
<!DOCTYPE html>
<html>
<head>
</head>
<body>
Name: <input type="text" id="name"><br>
Email: <input type="email" id="email"><br>
Course: <input type="text" id="course"><br>
<input type="button" value="Set Cookie" onclick="setCookie()">
<input type="button" value="Get Cookie" onclick="getCookie()">
<script>
function setCookie()
{
document.cookie = "name=" + document.getElementById("name").value;
document.cookie = "email=" + document.getElementById("email").value;
document.cookie = "course=" + document.getElementById("course").value;
}
function getCookie()
{
if (document.cookie.length != 0)
{
alert("Name="+document.getElementById("name").value+" Email="+document.getElementById("email").value+" Course="+document.getElementById("course").value);
}
else
{
alert("Cookie not available");
}
}
</script>
</body>
</html>
Output:
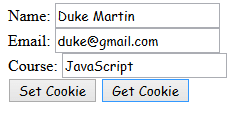
On clicking Get Cookie button, the below dialog box appears.
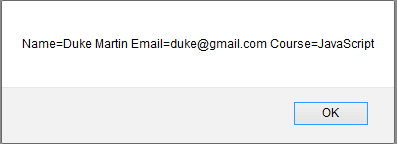
Here, also we can see that all the stored name-value pairs are displayed.
Leave a Reply